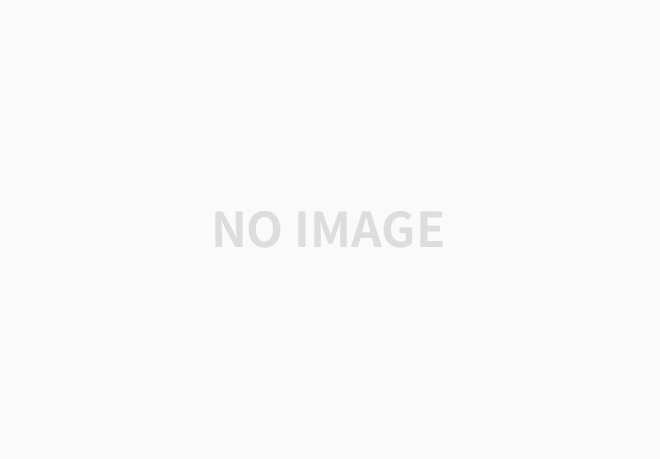
JSON(JavaScript Object Notation)
JSON이란 경량의 Data 교환 형식으로, '키:값' 형태로 이루어져 있다. 특히 인터넷 상에서 데이터를 주고 받을 때 자료를 표현하는 방법으로 알려져 있다. 실제로 API 요청을 하면 대부분의 서버에서 보내주는 데이터 형식이 JSON 형태이다. JSON의 기본 자료형은 수, 문자열, 불리안, 배열, 객체, null 등이 있다. 여기서 JSON 메시지 단위는 배열이나 객체이다. 텍스트로 이루어져 있어 사람과 기계 양 쪽에서 읽고 쓰기 쉬우며 JSON 파일의 확장자는 .json 이다.
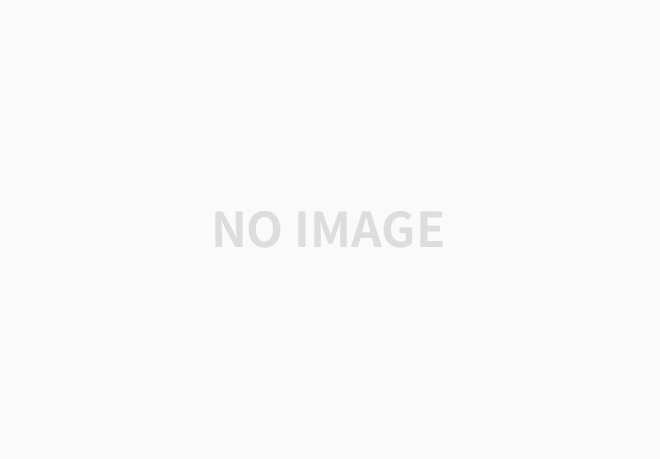
JSON.parse(), JSON.stringify()
JSON.parse()는 JSON 형태의 데이터를 받아와 javascript 객체로 바꾸어 주는 역할을 한다.
JSON.stringify()는 반대로 javascript 객체를 JSON 형태의 데이터로 바꾸어 주는 역할을 한다.
const movies = {
id: 1,
movie: 'LaLaLand',
director: 'Damien Chazelle'
}
console.log(movies);
const moviesStr = JSON.stringify(movies);
console.log(moviesStr);
const moviesPar = JSON.parse(moviesStr);
console.log(moviesPar);
Object {id: 1, movie: "LaLaLand", director: "Damien Chazelle"}
{"id":1,"movie":"LaLaLand","director":"Damien Chazelle"}
Object {id: 1, movie: "LaLaLand", director: "Damien Chazelle"}
movies라는 javascript 객체를 선언했다. 콘솔 창에 출력하면 Object 형식으로 나오는 것을 볼 수 있다. 그리고 JSON.stringify()를 통해 JSON 포맷으로 바꾸고 출력을 하면 객체 형식이 아닌 JSON 포맷으로 출력이 되는 것을 볼 수 있다. 그러한 JSON 포맷을 다시 JSON.parse()를 통해 javascript 객체로 바꾸면 처음과 같은 결과가 나오는 것을 볼 수 있다.
이번엔 javascript 배열을 JSON 포맷으로 바꿔 보자.
const movies = [
'LaLaLand',
'Whiplash',
'MadMax',
'Inception',
'Interstellar'
];
console.log(movies);
const moviesStr = JSON.stringify(movies);
console.log(moviesStr);
console.log(typeof moviesStr)
const moviesPar = JSON.parse(moviesStr);
console.log(moviesPar);
["LaLaLand", "Whiplash", "MadMax", "Inception", "Interstellar"]
'["LaLaLand","Whiplash","MadMax","Inception","Interstellar"]'
string
["LaLaLand", "Whiplash", "MadMax", "Inception", "Interstellar"]
다음과 같이 JSON 포맷으로 바뀐 moviesStr의 타입을 보면 string 형식으로 바뀐 것을 볼 수 있다.
'JavaScript' 카테고리의 다른 글
[JavaScript] 배열 내장함수 정리 (0) | 2020.05.15 |
---|---|
[JavaScript] spread 와 rest (0) | 2020.05.15 |
[JavaScript] 배열과 배열 내장함수 정리 (0) | 2020.05.13 |
[JavaScript] 객체(Object) (0) | 2020.05.12 |
[JavaScript] 화살표 함수 (0) | 2020.05.12 |
,