유튜브를 보다가 엘리님이 자바스크립트를 프로처럼 쓰는 팁에 관한 영상을 올리신걸 봤다. 마침 최근에 비슷한 주제의 블로그 글을 본 적이 있어서 이참에 같이 정리를 하려고 한다.
이 글에서 소개하는 팁들
1. 배열 비우기
2. 한 번만 실행시키는 이벤트 리스너
3. 'defer' 속성을 사용하자
4. 간결한 조건 체크(&&)
5. 범위 내의 랜덤 숫자
6. 빈 객체 검사하기
7. 배열 내 중복 원소 제거
8. 객체 복사하기
9. 삼항 연산자
10. Nullish coalescing 연산자(??)
11. Default Parameter
12. Logical OR 연산자(||)
13. Spread 연산자(...)
14. Optional Chaining(?)
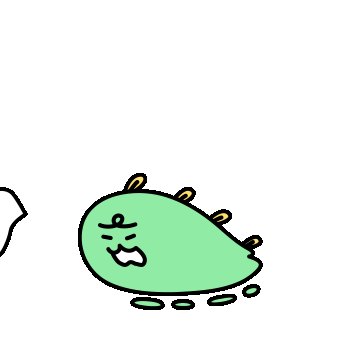
1. 배열 비우기
원소가 있는 배열을 빈 배열로 만들고자 할 때는 length에 0을 주기만 하면 된다.
let foo = ['morinana', 'gintoki', 'kagura', 'hina']
foo.length = 0
console.log(foo) // []
2. 한 번만 실행시키는 이벤트 리스너
이벤트 리스너는 바닐라 JS 뿐만 아니라 React, Angular와 같은 프레임워크에서도 많이 사용된다. 버튼 클릭을 예로 들자. 보통은 버튼을 누를 때마다 특정 로직을 실행되게 이벤트 리스너를 단다. 근데 만약 딱 한 번만 실행되게 하고 싶다면, 가능할까? 옵션 하나로 가능하다.
const button = document.querySelector('button');
button.addEventListener('click', () => {
console.log('한 번만 실행');
},{ once: true })
3. 'defer' 속성을 사용하자
자바스크립트 코드는 HTML 파일에서 script태그를 통해 다운로드되고 실행된다. 만약 head 태그 안에 script가 삽입되었다고 한다면, 어떻게 될까. 자바스크립트 파일이 간단하고 용량이 작으면 큰 차이를 못느끼겠지만 길고 엄청나게 클 경우에는 애플리케이션의 속도가 현저히 저하될 것이다. HTML 파일을 파싱할 때 script태그를 만나면 HTML 파싱하는 것을 멈추고 script를 실행시키기 때문이다. 그래서 보통 body 태그 맨 밑에 script 태그를 두곤한다.
하지만 defer속성을 사용하면 이럴 필요가 없다. 결론부터 말하자면 defer속성을 통해 script태그를 선언하면 head태그 안에 작성하든, body안에 작성하든, HTML를 파싱하면서 동시에 script태그를 통해 자바스크립트 파일을 다운로드 시킬 수 있다. 그리고 HTML 파싱이 끝나면 다운로드된 자바스크립트를 실행시킨다. 즉 script때문에 HTML 파싱이 멈추지 않게 되어 더이상 사용자가 빈 화면을 보지 않게 할 수 있다.
<script src="main.js" defer></script>
4. 간결한 조건 체크(&&)
어떠한 조건을 체크할 때는 if 대신 &&연산자를 통해 좀 더 깔끔하게 작성할 수 있다.
let condition = true
// 👎
if (condition) {
foo()
}
// 👍
condition && foo()
5. 범위 내의 랜덤 숫자
특정 범위 내에서 랜덤한 숫자를 간단하게 얻을 수 있다.
function randomNumber(num1, num2) {
return Math.random() * (num2 - num1) + num1
}
console.log(randomNumber(50, 100))
6. 빈 객체 검사하기
객체가 비어있는지 아닌지 검사할 때는 Object.entries() 메서드를 통해 쉽게 확인이 가능하다.
let foo = {
name: 'morinana',
occupation: 'actor'
}
let bar = {}
console.log(Object.entries(foo).length === 0) // false
console.log(Object.entries(bar).length === 0) // true
7. 배열 내 중복된 원소 필터링
중복 원소를 제거할 때는 Set을 사용하자.
let foo = ['morinana', 'gintoki', 'kagura', 'hina', 'gintoki', 'morinana']
console.log([...new Set(foo)]) // ['morinana', 'gintoki', 'kagura', 'hina']
8. 객체 복사하기
자바스크립트에서 객체는 참조 타입이기 때문에 =로 객체를 복사할 경우 원하지 않은 결과를 보게될 것이다. 올바르게 객체를 복사하기 위해서는 아래와 같은 3가지 방법 중 하나를 사용한다.
let foo = {
name: 'morinana',
occupation: 'actress'
}
// Object.assign() 메서드
const cloneFoo1 = Object.assign({}, foo)
// JSON
const cloneFoo2 = JSON.parse(JSON.stringify(foo))
// Spread 연산자
const cloneFoo3 = { ...foo }
9. 삼항 연산자
어떤 로직에서 두 가지 조건에 의해 결과가 나눠진다면 삼항 연산자를 사용하자.
// 👎
function getResult(n) {
let result
if (n > 5) {
result = '🐸'
} else {
result = '🦊'
}
return result
}
// 👍
function getResult(n) {
return n > 5 ? '🐸' : '🦊'
}
10. Nullish coalescing 연산자(??)
null이거나 undefined일 경우를 체크할 때는 ??연산자를 사용하자. ??연산자 기준 왼쪽 값이 null이거나 undefined일 경우 오른쪽 값을 채택한다.
// 👎
function printMsg(text) {
let msg = text
if (text === null || text === undefined) {
msg = '❌'
}
console.log(msg)
}
// 👍
function printMsg(text) {
const msg = text ?? '❌'
console.log(msg)
}
11. Default Parameter
함수 파라미터의 기본 값을 설정할 수 있다. 이때 이 Default Parameter는 undefined일 때만 적용이 된다.
function printMsg(text = '❌') {
console.log(text)
}
12. Logical OR 연산자(||)
||연산자 기준 왼쪽 값이 falsy일 경우 오른쪽 값을 채택한다. falsy에는 null, undefined 뿐만 아니라 0, -0, '', false 등도 포함된다.
function printMsg(text) {
const msg = text || '❌'
console.log(msg)
}
13. Spread 연산자(...)
객체의 프로퍼티를 업데이트 하거나 기존 객체를 그대로 사용하면서 새로운 객체를 만들때는 Spread 연산자를 사용하자. 여러 개의 객체를 한 객체로 묶는 것도 가능하다.
const movie = { name: 'lastletter', year: '2020' }
const detail = { actress: 'morinana', genre: 'drama', director: 'iwaishunji' }
// 👎
movie['genre'] = detail.genre
// 👎
const newMovie = {
name: movie.name,
year: movie.year,
actress: detail.actress,
genre: detail.genre,
director: detail.director
}
// 👍
const newMovie2 = Object.assign(movie, detail)
console.log(newMovie2) // { name: 'lastletter', year: '2020', actress: 'morinana', genre: 'drama', director: 'iwaishunji' }
// 👍
const newMovie3 = { ...movie, ...detail, actress: 'hirosesuzu' }
console.log(newMovie2) // { name: 'lastletter', year: '2020', actress: 'hirosesuzu', genre: 'drama', director: 'iwaishunji' }
14. Optional chaining(?)
특정 값이나 조건들의 값이 유효한지 여러개 나열해서 검사할 때는 Optional Chaining을 사용하자.
const nana = {
age: 19
}
const suzu = {
age: 23,
job: {
title: 'actress'
}
}
// 👎
function displayJobTitle(person) {
if (person.job && person.job.title) {
console.log(person.job.title)
}
}
// 👍
function displayJobTitle(person) {
if (person.job?.title) {
console.log(person.job.title)
}
}
displayJobTitle(nana) // undefined
displayJobTitle(suzu) // actress
참고
'JavaScript' 카테고리의 다른 글
[JavaScript] 드래그 앤 드롭 구현하기 (1) | 2021.09.15 |
---|---|
[JavaScript] 이벤트 버블링, 이벤트 캡처링과 이벤트 위임 (0) | 2021.03.31 |
[JavaScript] 비동기 처리의 꽃 async와 await (0) | 2020.11.10 |
[JavaScript] 비동기 처리를 위한 Promise (0) | 2020.11.10 |
[JavaScript] 부분 문자열 추출하는 방법 (0) | 2020.10.27 |